Ok guys, here's what my questions have been about.

Basically, a scripted elevator solution. I'm posting now because, with serious help from you guys,

it is actually at a working proof-of-concept stage.
The idea is this:
- a multifloor building (since there aren't too many single-story buildings with elevators)
- a room in the building that is identical on each floor (the "elevator") that is basically an elevator shaft, but with floors.
- a trigger in "front" of the doors to the room that is the "call elevator" trigger - really just a script with a delay before it triggers the door animation
- a trigger that is the same size as the room (will take some trial and error) that is the elevator script
Here's a screenshot of the idea for the setup of the elevator trigger.

The mission designer will have two choices. He can put the number of floors in the trigger execVM and the script will automagically generate the heights of the floors (for the hotel I'm using to test, a factor of *3.5 seems to work well). There is also a commented guide in the script in case someone has a building model with inconsistent floor heights (like a tall atrium on the first floor or something).
The "elevator" script generates the addactions.

// Elevator script 01d
// by Trexian for JTD (with help from OFPEC)
//
// This is triggered when the player moves into the trigger that
// defines the "inside" of the elevator.
// hint "started";
// sleep 2;
// variable defines
_numFloors = _this select 0;
addedActions = [];
// Loop from 1 to _numFloors - used as index for addedActions array and floor selection display.
for "_i" from 1 to _numFloors do
{
_index = player addAction [format["Floor %1", _i], "JTDFloorSelect01c.sqf", _i, 10, false, false, ""];
addedActions = addedActions + [_index];
};
/* ----------------Manual version
You must define how many floors you have. Notice the third parameter is the floor number, also.
elevAddAct1 = player addAction ["First floor", "JTDFloorSelect.sqf",1,10,false,false,""];
elevAddAct2 = player addAction ["Second floor", "JTDFloorSelect.sqf",2,10,false,false,""];
elevAddAct3 = player addAction ["Third floor", "JTDFloorSelect.sqf",3,10,false,false,""];
elevAddAct4 = player addAction ["Fourth floor", "JTDFloorSelect.sqf",4,10,false,false,""];
elevAddAct5 = player addAction ["Fifth floor", "JTDFloorSelect.sqf",5,10,false,false,""];
*/
exit;
Then the "transporter" script moves the z position (right now just of the player).
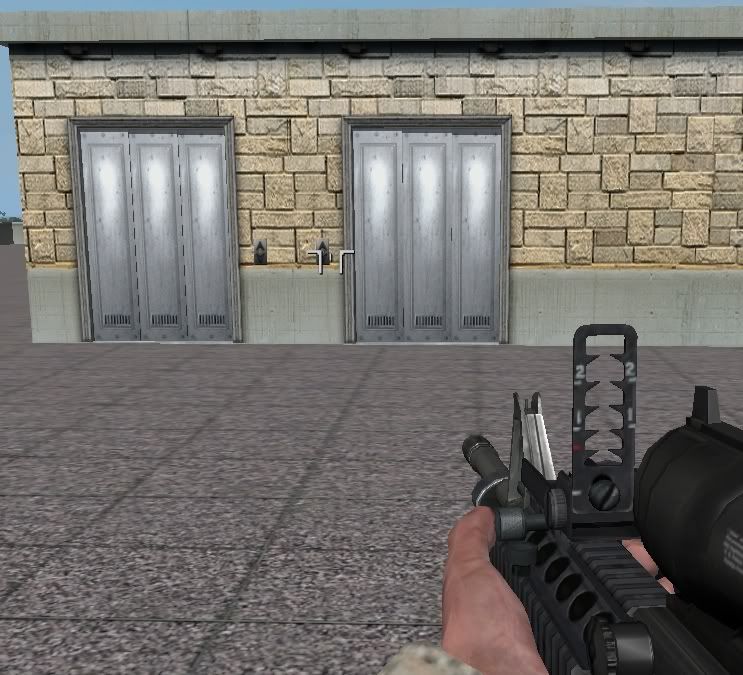
// Elevator floor transporter
// by Trexian for JTD
// This is called by the addactions and actually transports the player.
_flrSel = _this select 3; // the floor selected by the addaction
_Here = getpos player;
//this section gets the xyz of the player to keep xy constant
_Plx=_Here select 0;
_Ply=_Here select 1;
_Plz=_Here select 2;
_Newz = 0; // sets the scope and beginning value of the new height
_flrSel = _flrSel -1; // subtracts one from the floor selected so the math works
// Easiest way to do it, but have to change string to numeric
_Newz = _flrSel * 3.5;
//--------------- Manual way to set the height of the floors
/*
_Newz = switch (_flrSel) do // sets the switch to define the new height based on which addaction
// new height = the value in the "case" that corresponds to the selected floor
{
case 1:
{
0.0014 // this is the z height for the first floor
};
case 2:
{
3 // this is the z height for the second floor
};
case 3:
{
6.75 // and so on
};
case 4:
{
10.5
};
case 5:
{
14.25
};
case 6:
{
14
};
};
*/
player setpos [_Plx,_Ply,_Newz]; // sets the player same xy, new z
hint "trigger door animation";
sleep 2;
hint "remove actions"; // removes the actions since the player is at selected floor
{ player removeAction _x } forEach addedActions;
addedActions = nil;
sleep .5;
//--------------- Manual version, you have to remove the actions
// for each elevAddAct#, you'll need a:
// player removeAction elevAddAct#;
exit;
I remove the addactions after arriving at the desired location for now.
Then, the on deactivation script to remove the addactions if the player gets out of the elevator/off the trigger:
// Elevator remove action script
// by Trexian for JTD
// removes addactions when player moves off of trigger
hint "remove actions";
{ player removeAction _x } forEach addedActions;
addedActions = nil;
sleep 1;
hint "end";
exit;
/*
//--------------- Manual version, you have to remove the actions
for each elevAddAct#, you'll need a:
player removeAction elevAddAct#;
*/
exit;
Moving forward, there are still many things to do:
1) generate list of units "in" the elevator/on the trigger into an array, and change all of their z positions;
2) make/get a model with the right kind of elevator "shaft" (might just modify one of the BI models);
3) properly animate the doors;
4) have the script be able to trigger the correct door;
5) get it to work in MP also.
Yeah, I'm probably only about 10% done.

Any advice, warnings, insight will be greatly appreciated!
And thanks for all the help getting this n00b this far.

PS - Ok... I have no idea why it is putting the whole post in a code bracket. :doh:
PPS - Whew - worked out the whole code tag thing.
